Introduction
Specify is a .Net testing library that builds on top of BDDfy from TestStack. While BDDfy is primarily intended for BDD testing, it is beautifully designed to be very easy to customize and extend. Specify provides a base test fixture that extends BDDfy with additional features, such as automatic creation of the SUT, using auto-mocking or your IoC container, and logging with your logging framework.
When I first started using BDDfy for acceptance testing, I would use a different framework for unit testing, but I didn't like the context switching between different frameworks, syntaxes and testing styles. Specify provides a base fixture which gives a consistent experience for all types of tests (or specifications). Why not have the fantastic BDDfy reports for all of your different test types?
Overview of Features
- Tests use a context-specification style, with a class per scenario.
- SUT factory with pluggable auto-mocking or Ioc containers. There is transparent built-in support for NSubstitute, Moq, and FakeItEasy.
- Tests can be resolved from your IoC container. There is built-in support for the Autofac container.
- BDDfy Reports are produced for all of your test types
- Specify uses LibLog for logging, a logging abstraction which provides support for NLog, Log4Net, EntLib Logging, Serilog and Loupe, and allows your users to define a custom provider if necessary.
Sample Scenario
Just write a simple C# class using the Gherkin Given When Then
syntax that inherits from the ScenarioFor base fixture. No pesky attributes either!
public class DetailsForExistingStudent : ScenarioFor<StudentController>
{
ViewResult _result;
private Student _student = new Student { ID = 1 };
public void Given_an_existing_student()
{
Container.Get<ISchoolRepository>()
.FindStudentById(_student.ID)
.Returns(_student);
}
public void When_the_details_are_requested_for_that_Student()
{
_result = SUT.Details(_student.ID) as ViewResult;
}
public void Then_the_details_view_is_displayed()
{
_result.ViewName.Should().Be(string.Empty);
}
public void AndThen_the_details_are_of_the_requested_student()
{
_result.Model.Should().Be(_student);
}
}
This produces great reports, as powered by BDDfy. Note that, it specifies "Specifications For: StudentController" rather than the normal BDDfy user story. This makes more sense for unit specifications. You can still apply user stories if you want to though.
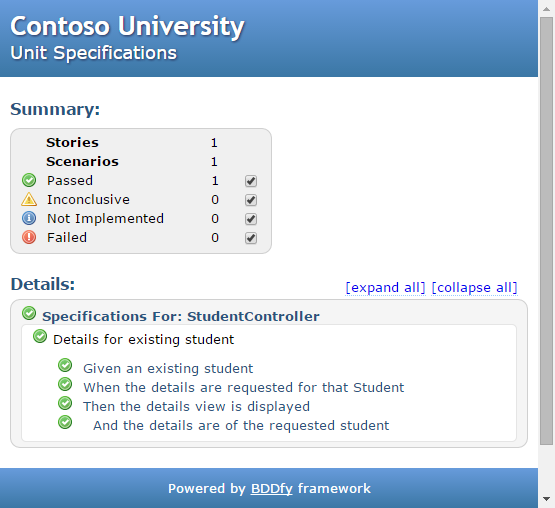
Updated less than a minute ago