Architecture
Your testing framework discovers the Specify
method on your Scenario class (due to the attribute on the method, or the convention in the case of Fixie) and executes it. This method passes the Scenario class to Specify, which configures it to run and then passes it to BDDfy to execute. BDDfy uses reflection to discover all of the relevant methods and executes them in the correct order, finally producing various reports for all of the Scenarios in this test run.
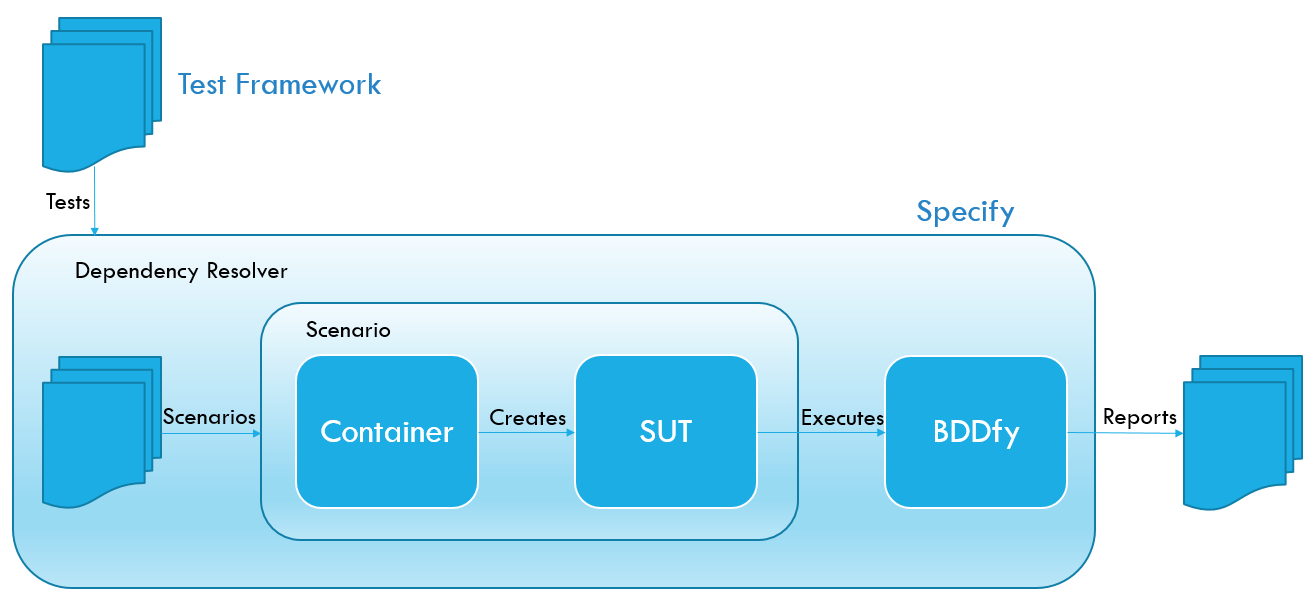
Specify resolves the Scenario from the Dependency Resolver, which is an IoC container. It sets the Container property of the Scenario to be an auto-mocking container or a child IoC container, meaning that you can override container registrations on a per scenario basis and the following Scenario will be reset with a fresh new container. The Container is used to create the SUT (the thing that is being tested) and to provide mocked or real dependencies for use in the Scenario.
Dependency Resolver
Specify creates a single Dependency Resolver for the entire test run. The Dependency Resolver is an IoC container which contains all of the Scenario classes and all of the dependencies for your application. If you are using an IoC container in your application, then you would probably use the same container and configuration for the Dependency Resolver, perhaps with some mocked out for testing purposes.
Why is this useful? Being able to resolve your Scenarios out of your container makes writing integration tests easy, as you can use the exact same container you use to compose your application normally to compose it for your integration tests. As the Scenario will be resolved out of your container, you can get the components you want to test injected right into your Scenario's constructor.
Container
The Container can either be an IoC container or an auto-mocking container. If you are using an IoC container, then Specify will provide a fresh new child container for each Scenario. This means that you can override registrations on a per Scenario basis and remain side-effect free from other Scenarios.
If you are using auto-mocking then Specify has transparent built-in support for NSubstitute, Moq, and FakeItEasy auto-mocking containers. Just add a reference to one of these projects and Specify will detect it and use the relevant adapter.
If no mocking framework is referenced then Specify will default to an Autofac-based IoC container. Just add an Autofac module, which Specify will automatically detect and register your dependencies.
Alternatively, to use a particular mocking framework or IoC container in your tests you just have to implement the Specify IContainer
interface.
SUT (System Under Test)
SUT, or System Under Test is a term from the XUnit Test Patterns book, and refers to the class that is being tested. Each Scenario has a SUT
property, which is instantiated for you by the auto-mocking or IoC container. You can override this too, if you want.
The SUT property is lazily initialized the first time that you call it's Get property. You tell Specify what your System Under Test is going to be by specifying it as the type parameter when you derived from the ScenarioFor<T>
base fixtures. Whatever you specify for T
becomes your System Under Test.
Updated less than a minute ago